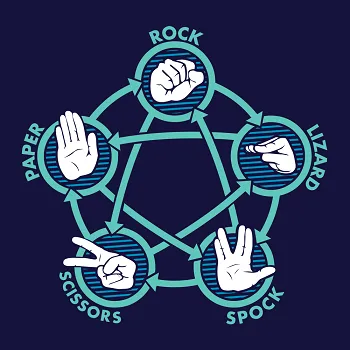
Pentaskill is the game I'm making for the Steem blockchain.
It is a zero-sum peer-to-peer gambling dapp essentially modeled around the basic game of rock/paper/scissors (or should I say Block Paper Scissors?).
Gameplay
In any case, each skill in the game counters 2 other skills. Each skill has a "soft counter" that currently is set to an arbitrary 100 damage. The other is the "hard counter" that does 200 damage. Currently, the default HP of avatars is 500, so if a player takes 500 damage they lose the game and have to pay the winner the bet amount (if any).
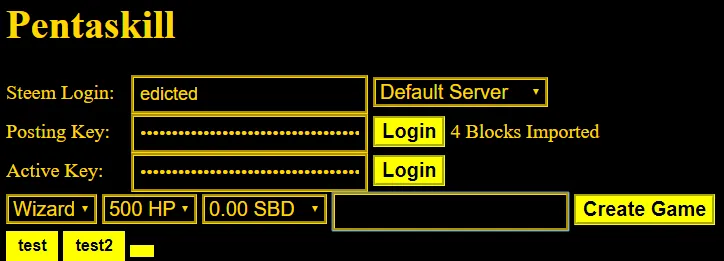
Remember that I'm new to both the Steemit API and Javascript. I've already bitten off more than I can chew so I currently have to use the super basic Document Object Model (DOM) for the frontend API at the moment. As I've stated before, as long as the backend isn't finished I have no reason to really worry about the frontend. More on that later.
Regardless, it all starts by entering your Steem username / posting key. When you click the login button it connects to Steemit's node (or whatever other node that can run this code), and requests block information to start streaming to your computer. This turns your computer into somewhat of a proxy, trusting the information that you are receiving from the node you've connected to.
My original vision of this game was that it should still technically work without a centralized database node (unlike every other 'dapp' out there). By using a Steem node for input/output, we should be able to get all the information needed and be able to respond in a "timely" manner. I put timely in quotes there because streaming blocks from a node seems to have a delay of around 6 seconds at best, and if you want to import immutable blocks you have to wait a full minute (not a viable option).

Create a game.
At first I was trying to do game creation using traditional lobbies. The "host" of the game would create a lobby and other players would be able to join that game. This made the whole process overly complicated and prone to attack.
Instead, in order for a game to be legitimate, both players have to be specified beforehand. This is the equivalent of inviting them to a game. If you don't know who you want to invite to a game, players simply create one with player 2 as an empty string. Inside my code I call this a ping
because player 1 is pinging the Steem blockchain asking any of the proxies that are listening if they want to invite them to a game with the variables specified. I changed it to work this way just recently and it's simplified a lot of the complexity out of what I was trying to accomplish.
Variables
Right now you choose what version of the game to use, how much HP you start out with, how much you want to bet, and who your opponent is. Currently, there is only one version of the game. I'm focusing on the "Wizard Battle" skin right now. It's crap, but then again it's supposed to be crap, by design. I'm trying to incorporate decentralized development into my project. This is simply proof-of-concept to show that it can work at the base level.
So I've got the 5 skills out there (fire, lightning, water, wind, earth). When you hover over them they turn into the gif version.
I've scaled them all down to 100x100 pixels just for consistency.
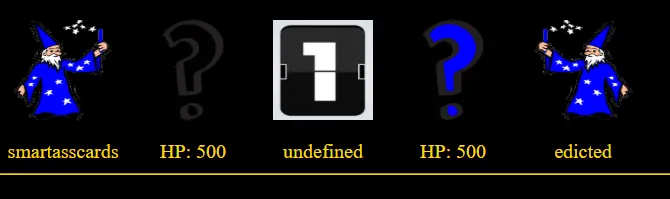
When the question marks light up it means that the player has chosen their skill and it is being stored in secret on the blockchain in the form of an SHA-256 hash (Bitcoin's hashing algorithm).
function obfuscate(skill, game){
// SHA256 hash skill choice combined with temporary password.
let password = Math.random().toString() + Math.random() + Math.random()
+ Math.random() + Math.random() + Math.random() + Math.random()
game['hero_password'] = password
return sha256(skill+password)
}
This hash is created by taking the skill and appending a temporary password (nonce: number only used once) to it and then applying the SHA-256 function. Currently we see that the password is created by stringing 7 Math.random() together. This is technically not secure because random numbers are based on time and you can reverse engineer them, but I'm not too worried about that at the moment.
After both players have cast their "swings" (skills) in the form of an obfuscated SHA-256 hash, both clients know that it is safe to reveal the password (nonce) to the network.
Each client then reverse engineers the password back into what skill was chosen (there are only 5 possibilities).
function decipher(password, hash){
// Use temporary password provided by villain to confirm skill selection.
// returns 'skill1' || 'skill2' || 'skill3' || 'skill4' || 'skill5'
for(let s = 1; s <= 5; s++){
let skill = 'skill' + s
if( sha256(skill+password) === hash ){
console.log(skill);
return skill
}
}
console.error('skill not found in decipher()');
return undefined
}
Once the client knows what skill the opponent has chosen the round can be resolved with damage.
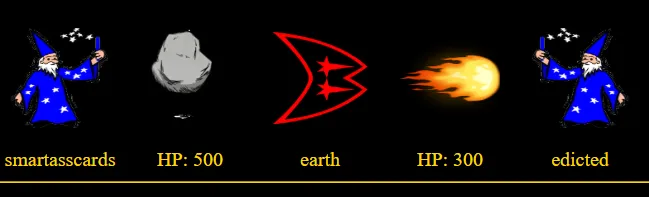
This is an example placeholder for resolved damage.
It shows that earth hard counters fire and player2 takes 200 damage.
Ideally a custom animation would play showing earth countering fire and perhaps a flaming boulder hitting the player2 wizard for heavy damage. I assure you I don't have time for that (or the ability) :D.
Decentralized development.
This is where the Steem community comes in. Anyone will be able to create a gif and submit it to the network. That user would claim ownership over their work and be able to charge other players a small amount to use the version of the game that they've created. This would allow me to essentially focus on the backend while allowing me to outsource the frontend to the community in the form of paying jobs.
Bounties
I can also imagine a situation where bounties are placed on jobs that users in the Pentaskill network want done. For example, perhaps I want 5 different gifs of the wizard avatar getting hit by each of the 5 abilities. I could put a bounty on that job and all the upvotes and donations that bounty received would just add up until someone took the job. Obviously it's a bit more complex than that because then you have to worry about quality of work and denying someone the money who did a bad job, but you get the idea.
Hacked version?
For the most part, I imagine this game to be fully open source and pretty easy to hack. We can't really force people to pay for these low-rent GIF skins. However, I imagine a simple honor system should work well enough starting out. How many people are really going to seek out a hacked version of the game when their posting/active key security is on the line? Seems like a silly prospect. Also there are the bragging rights that go along with proof that your account supported the network via atomic-swap money transfer directly on the blockchain. It will be quite easy to prove who owns what legitimately just by looking up the transactions to the owners of the "skins".
Here's an example of me finding a death animation GIF on Google Images, reversing the contrast, and then adding extra frames to it.
Who owns what and how?
So lets say someone creates a GIF and they want to claim ownership of it. There would be some custom JSON for that... not even close to programming that in any time soon unfortunately. It would have to be approved in some way (probably by me to start and perhaps with a governance coin once I have a chance to automate the approval process). I might even use SteemLeo as the governance coin seeing as how huge of a stake I have there.
In any case I think when a GIF (or group of GIFs packaged into a skin for the game) gets approved it would start out as having an infinite supply and the price would be determined by the owner. From here, the owner could execute a function that would reduce supply from infinite to a limited supply (perhaps in the form of non-fungible tokens; one coin equals one skin of the given type). This would allow skins to have scarcity, and if the owner sold them all the supply would run out and prices would fluctuate (assuming on the SteemEngine platform).
Speaking of SteemEngine, the only way this game can become truly decentralized if it eventually becomes enforced by smart-contract. I imagine SteemEngine will be the first place this feature will become available to me.
The network could even have a rule that all skins older than a certain age (say one year) become public domain and are owned by the network. This means to buy them you'd have to send Steem directly to @null, increasing the scarcity of liquid Steem coins.
Skins could obviously also be donated to the network in this way, perhaps increasing the reputation of devs that choose to provide this kind of value to the network pro bono.
How to enforce payment.
Barring the use of smart contracts, I can see two possible ways payment from the loser to the winner can be enforced. They are both not great.
The first way will just be through the honor system. You only play against accounts that have the money to pay up and you trust to do so. Any loser than doesn't pay will have their account "burned" by the network and everyone will know not to play with them again in the future until the matter is resolved. However, payment should be fairly automatic otherwise and users won't have to think about it unless there is a problem. This feature somewhat works already.
The second way to enforce payment is through a decentralized escrow service. Players who want to use this service would send out a ping to the network asking active escrow accounts to respond. Any account can be an escrow account. The host of the game chooses a trusted third party (or themselves) to be the escrow. Player 2 can either accept the escrow or offer an invite using a different one. The escrow account monitors the game and pays the winner. Escrows will be allowed to rake a fee if they want to or get tipped, so it could be a good way to fund projects. Of course there will likely always be someone available willing to do it for free so that's also a consideration.
Obviously both these solutions are 100% inferior to a smart-contract solution, but the complexity of such a thing is also not worth fussing with at the moment. As far as I know, the tech just doesn't exist yet, and if it does I need to learn it.
second layer scaling solutions
The worst thing about this game is how laggy it is. There's a 6 second delay between a block being posted and that block being uploaded to you from the Steemit server. I'd say it takes about 15 seconds to complete a single round because the skill being used has to be hidden and then revealed in two separate sequential operations.
This lag has really got me wondering if it's possible to currently sign these transactions and share them peer to peer rather than over the blockchain itself. A solution like this could save network resources and reduce lag time from a latency of 1500 ms to under 100 (a tenth of a second). You'd only have to go back to the main chain to transfer the money or if there was a dispute (like someone trying to cheat). Again, I'm getting way ahead of myself here. Right now I'm just focusing on getting it working. It doesn't. :(
yet...
Conclusion.
Well I've been talking about Pentaskill on and off for a while now but haven't really shown what it looks like on my screen at the moment. Didn't really want to share it because it's the first thing I've done in both JavaScript and the Steemit API, so it's obviously hot garbage at the moment. However, I have big dreams.
Honestly, this could turn into the most decentralized gambling app in the world. I'm not trying to capture value over here. What I'm trying to make is a pure peer to peer system where no one takes a profit from the winnings. Every other gambling app in the space attempts to capture value for the developers. Not only does this project shy away from that idea, but I am also trying to incorporate decentralized funded development of the frontend by implementing GIF ownership consensus within the network.
Hopefully something can come from this but if not it was still a valuable learning experience.
Although trying to learn JavaScript, Steemit API, GIMP image editing, Node.JS and GitHub all at the same time has been a bit foolhardy.